Published by Jady on 5/1/24, 2:54 PM
I've been mainly working on the Echidna Engine editor. Currently everything is very grayboxed, but slowly but surely
we're adding features that will make development way more streamlined than Unity or Godot! One of these features is
"favorite fields", so you have a place to put all your most important data so the display doesn't get all clogged
up!
Published by Jady on 1/30/24, 12:59 PM
If you've been hanging around the server lately, you might've noticed a new SBEPIS channel: Echidna Engine. Echidna
is going to be our in-house engine that SBEPIS will be moved to, off of Unity. I've been struggling a lot with how
Unity's systems operate, between its limited layer and tag systems, its inaccessibility for planetary geometry, and
just how dang slow it is, especially with their physics system. So over the past couple weeks I've been
writing my own game engine from scratch in C# using OpenGL and BepuPhysics, and we're calling it Echidna after the
Denizen of Space.
The primary immediate improvement over Unity is its speed. Unity only runs the majority of its code on one thread at
a time, because it uses Component architecture, which means that entities contains components that do things.
The only place Unity can use multiple threads at once is through its job system, which has Entity Component
System (or ECS) architecture, where entities have components that don't do anything, but the world
contains systems that can run on many components at once. Echidna fully uses ECS architecture to take full
advantage of multithreading, and this can be applied to any system that needs it. The entire engine code only uses 4
files, World
, Entity
, Component
, and System
. Everything else is
just sticking components on entities adding systems to the world.
If you're interested in poking through an ECS game engine, all the code is Free and Open Source on my GitHub.
Published by Jady on 5/17/23, 5:34 PM
We have these fancy new outlines now! They appear when an object is still in the process of being captured.
I've been suffering most of the day trying to write my own renderer features, but then I found out that
everything I want to do is actually way easier in the current version of unity than all the old tutorials
I was using. You can just make a shader that runs on the finished image when a camera finishes rendering.
If found a tutorial for this outline shader that takes the difference between the depth of a pixel and the depth
of the surrounding pixels, which gives you an outline. I made a second camera that's parented to the main camera
that uses a separate renderer asset with the new shader on it (set to a specific outlines layer), then hooked that
up to a texture that I load onto the main camera through another shader on the main renderer asset when the game
starts.
Here are those shaders, the outline and the texture overlay. Thanks to Game Dev Guide and this video or the outline shader, although the rest of
the video uses the old bad built-in Unity renderer.
Published by Jady on 5/16/23, 8:07 PM
Remember kids! Sometimes it's way easier to just have an arbitrary list of little specific things instead of
one big thing that has all your use cases! For the Hashmap Deque that I just released, I needed all the settings for
laying cards out in a line, and also the settings for choosing your hash function. After a few hours of trying to
stuff both of them together, I just made them separate and stuck them in a list. It's much easier to use now,
although if something doesn't get set up right it now throws an error in-game instead of just not letting me play
(which is usually better).
Also if you're making video recordings, make sure your encoder actually has the right settings :/
Published by Jady on 5/8/23, 1:07 AM
Today's super technical blog post: Interface inheritance!
Interface: an object type that stores no data, only manipulates it, and can therefore be used with other interfaces
to make a complicated object type
C# has a neat trick where you can override one of the functions of an interface so that nothing else can access it!
This was really helpful for reworking the deque rulesets, because each rule was split into two parts:
one for a generic data type, and one for the data type that the deque actually uses. But also, those rulesets
weren't actually interfaces because they stored data. I thought that was cringe! So I stuffed all of the data into
another class I was already using, SingleDeque, which represented a single "layer"
(like Array but not like Queuestack). So then with the interfaces created, I could hide the generic wishy washy
versions of all the rules so that deques can only access the specialized versions.
So now it's easier to write deques without breaking anything!
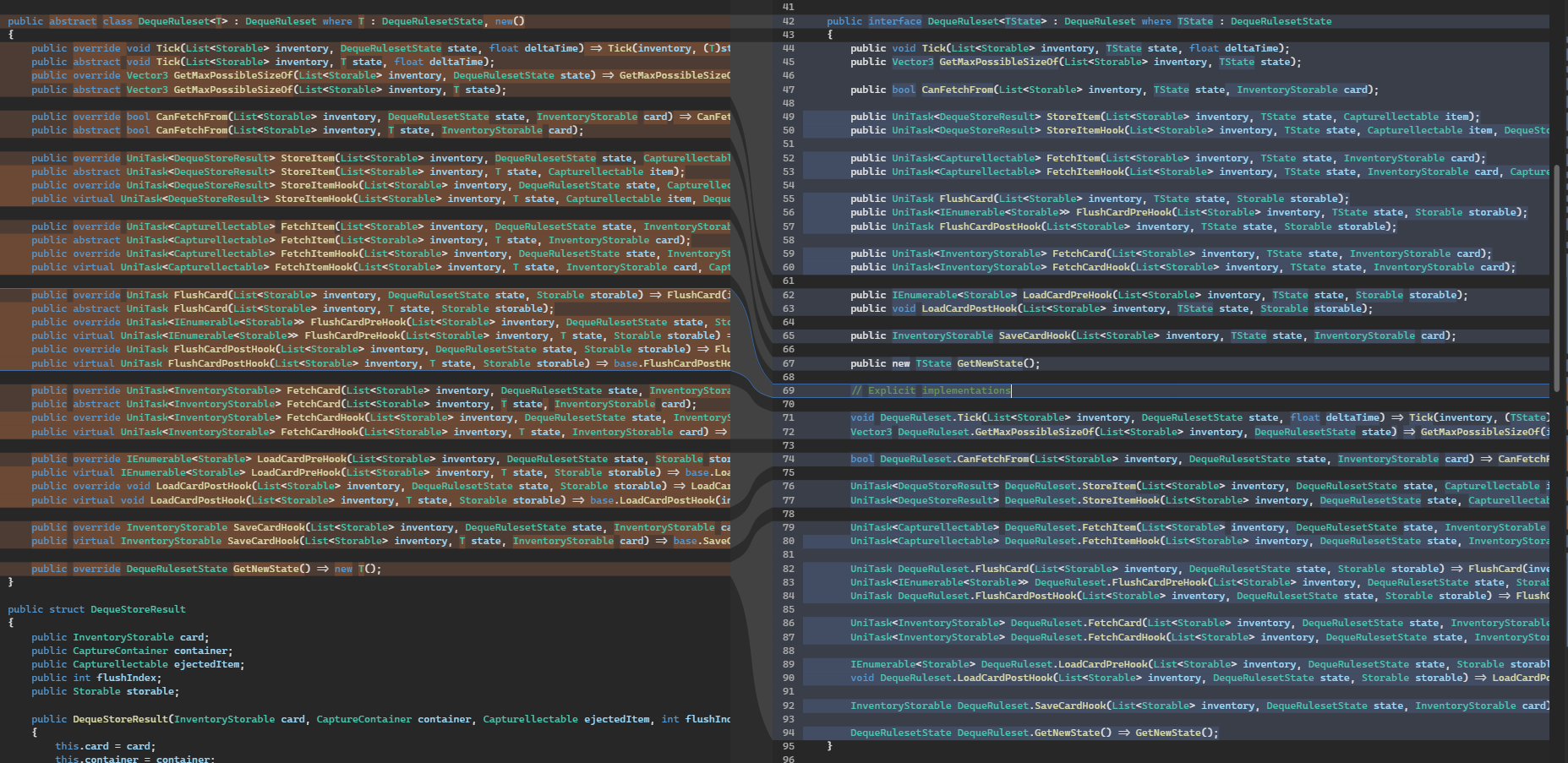
The other big thing I did today was create special objects to manage how the cards were laid out on the board.
Previously, I had to copy and paste all the layout code from Stack to Queue since they pretty much are the same
thing visually. Now each layout type gets its own object.
But then there was an issue in that each deque had its own type of data to use with the layouts,
and you can't really have one function accepting all sorts of different data. So the obvious answer was to ignore
the data completely and just use interfaces! So now all the data has been turned into a collection of "features":
one for keeping track of direction (which Stack, Queue, and Array use), one for keeping track of time (which Cyclone
and Array use), one for keeping track of flipped cards (which Memory uses), etc. Then I can just have each deque
hold its own data, and only give the layouts the interfaces that manipulate that data! So now a WobblyLayout just
knows it needs something for direction and time, but it doesn't care about the data behind it, so I can just give it
the interfaces Array has, and it'll just accept that!